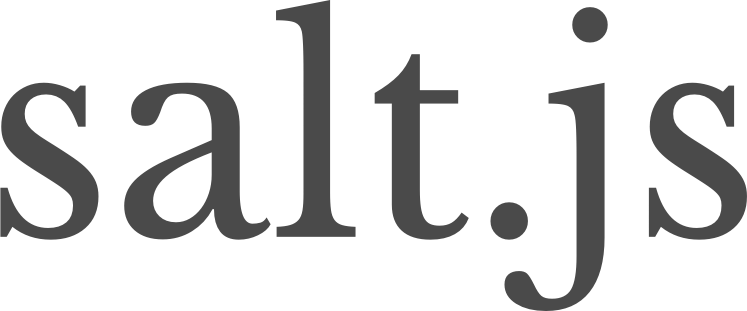
I made a tiny dom selector library called Salt.js.
It uses a regular expression to map different queries you pass through it to their native get functions. The reason I don’t just use querySelectorAll
for everything is because it is slower than the native get commands. See this jsperf test.
Yes, I see that the mapping is slower for newer versions of Chrome. But, almost every other browser and device is slower using querySelectorAll
over the mapping method. Also keep in mind the regex used in that example is much more complicated than mine.
Here are some examples of how you would use the library:
Salt.js Examples
// get by id
$('#iddiv');
// get by class name
$('.classdiv');
// get by element name
$('@namediv');
// get by element tag name
$('=div');
// get element using querySelectorAll
$('*div div.inside');
// getAttribute of name
$('#iddiv').getAttribute('name');
// getAttribute of name from nodelist
$('.classdiv')[0].getAttribute('name');
Check out the library on Github.
Update
Looks like there are a bunch of better ways to make this smaller! I’ve updated the github to reflect the new libraries. I have also added a jsPerf test.
James Doyle
I'm a full-stack developer, co-organizer of PHP Vancouver meetup, and winner of a Canadian Developer 30 under 30 award. I'm a huge Open Source advocate and contributor to a lot of projects in my community. When I am not sitting at a computer, I'm trying to perfect some other skill.